728x90
1. PThread란?
POSIX Threads, PThread는 병렬적으로 작동하는 소프트웨어의 작성을 위해서 제공되는 표준 API이다.
2. pthread_create()
pthread를 생성하기 위해서는 pthread_create()함수를 사용한다.
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void *), void *arg);
- thread_t : 스레드를 구분하기 위한 ID
- attr : 스레드를 생성할때 필요한 속성 값
- start_routine : 스레드로 해야할 일을 정의한 함수
- arg : 세번째 인자에서 사용한 start_routine 함수로 넘겨주는 값
함수의 호출이 성공하면 첫 번째 인자에 pthread_t형의 현재의 스레드에 대한 ID를 설정하고 0을 반환한다.
3. pthread_join()
스레드는 종료할때 까지 대기해야 한다. 서브 스레드가 실행되고 있는 때는 메인 스레드가 종료되지 않도록 해야하는데 이때 pthread_join() 함수를 사용한다.
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
- thread : pthread_create()에서 설정된 스레드의 ID
- **retval : start_routine에서 반환되는 값을 받기위해 사용
4. 예제
컴파일 시 -lpthread를 추가한다.
ex) g++ -o thread thread.cpp -lpthread
#include <iostream>
#include <cstdlib>
#include <unistd.h>
#include <pthread.h>
using namespace std;
void* function_A(void* num)
{
int i = 0;
while (true)
{
cout << "funcA i = " << i << endl;
i++;
sleep(1);
}
}
void* function_B(void* num)
{
int i = 0;
while (true)
{
cout << "funcB i = " << i << endl;
i++;
sleep(2);
}
}
int main()
{
pthread_t ptA, ptB;
cout << "create Thread A" << endl;
pthread_create(&ptA, NULL, function_A, NULL);
cout << "create Thread B" << endl;
pthread_create(&ptB, NULL, function_B, NULL);
pthread_join(ptA, NULL);
pthread_join(ptB, NULL);
return 0;
}
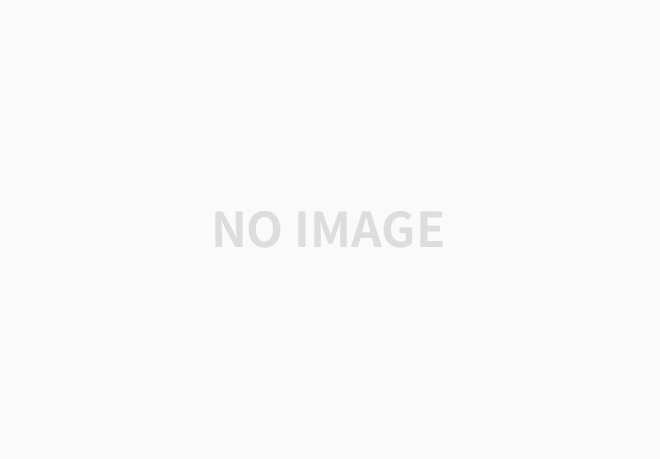
728x90
'Programming > Raspberry Pi' 카테고리의 다른 글
라즈베리파이 외부에서 VNC 접속하기 (3) | 2019.11.03 |
---|---|
라즈베리파이 한글 입력하기 (0) | 2019.10.31 |
라즈베리파이 MariaDB 설치하기 (0) | 2019.08.28 |
라즈베리파이에서 인체감지센서(SIS612P) 사용하기 (2) | 2019.08.20 |
라즈베리파이 motion 사용하기 (2) | 2019.08.10 |